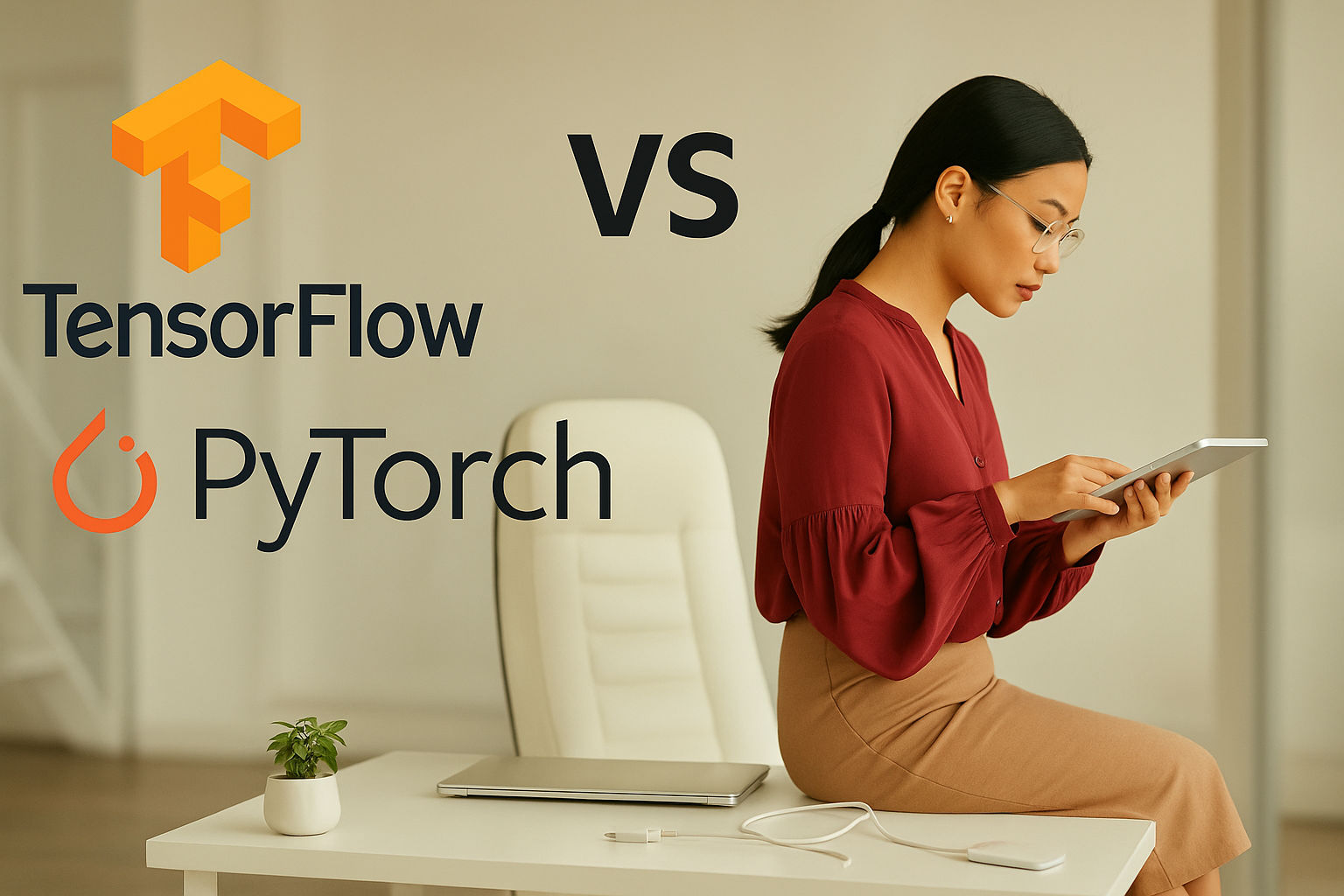
TensorFlow vs PyTorch: Choosing a Deep Learning Framework
An in-depth analysis of TensorFlow and PyTorch for deep learning projects.
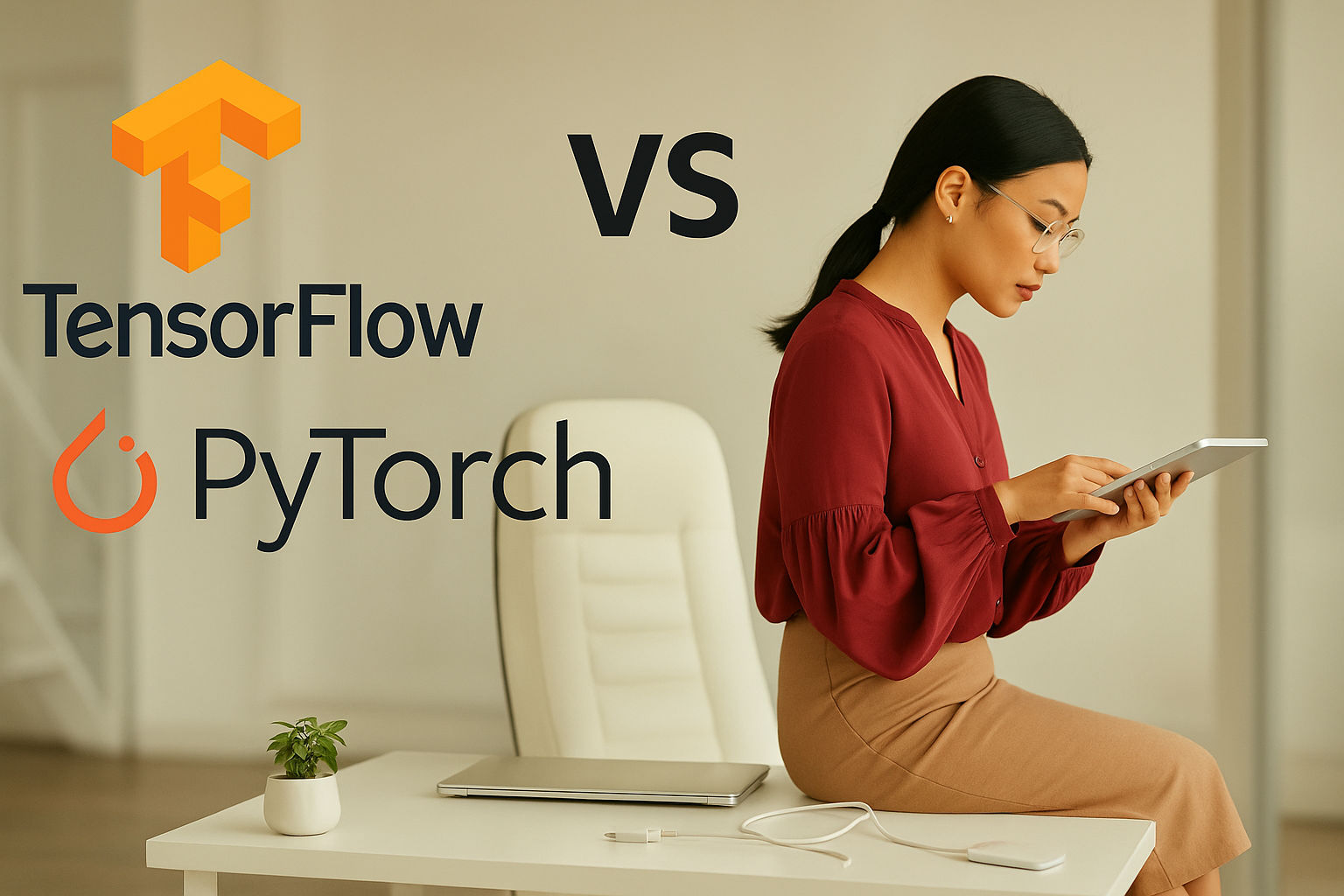
Table of Contents
TensorFlow and PyTorch are two of the leading frameworks for deep learning, each offering unique features and capabilities. This post will explore their differences, strengths, and weaknesses to help you choose the right framework for your project.
Introduction
TensorFlow, developed by Google, and PyTorch, developed by Facebook (Meta), are both open-source frameworks widely used in the field of deep learning. While TensorFlow is known for its production-ready capabilities, PyTorch is praised for its simplicity and ease of use.
Deep learning seeks to develop human-like computers to solve real-world problems using special brain-like architectures called artificial neural networks. Both frameworks provide tools to build, train, and deploy these neural networks for various applications.
TensorFlow was first released in 2015 and has evolved significantly with TensorFlow 2.0 introducing eager execution and a more intuitive API. PyTorch, released in 2016, has gained popularity in research communities due to its dynamic computational graph and Pythonic nature.
Framework Overview
TensorFlow is an open-source deep learning framework for Python, C++, Java, and JavaScript. It can be used to build machine learning models for a range of applications, including image recognition, natural language processing, and task automation. TensorFlow is widely applied by companies to develop and automate new systems, with its reputation built on distributed training support, scalable production, and deployment options for various devices including mobile platforms.
PyTorch is an open-source deep learning framework that supports Python, C++, and Java. It is commonly used to develop machine learning models for computer vision, natural language processing, and other deep learning tasks. PyTorch has gained popularity for its simplicity, ease of use, dynamic computational graph, and efficient memory usage.
At their core, both frameworks work with tensors—multi-dimensional arrays that store and process data. The fundamental difference lies in how they handle these tensors and execute computations.
Ease of Use
PyTorch is often considered easier to learn and use, especially for beginners. Its dynamic computation graph and Pythonic nature make it intuitive and flexible. TensorFlow, while more complex, offers a comprehensive suite of tools for building and deploying machine learning models.
TensorFlow 2.0 has significantly improved its usability with the Keras API as its high-level interface, making it more accessible to beginners. However, PyTorch's design philosophy of being Pythonic and straightforward still gives it an edge in terms of ease of use and learning curve.
Key usability differences:
- PyTorch: More intuitive for Python developers, feels like standard Python
- TensorFlow: More structured approach with multiple abstraction levels
Performance
Both frameworks offer excellent performance, with TensorFlow often preferred for large-scale production environments due to its optimization capabilities. PyTorch, however, is highly efficient for research and prototyping.
Performance benchmarks typically show that both frameworks are comparable in terms of training speed and inference time. TensorFlow may have a slight edge in production environments, especially when optimized with TensorFlow Serving, while PyTorch excels in dynamic neural networks and research settings.
Performance considerations:
- TensorFlow: Better optimization for distributed training across multiple GPUs/TPUs
- PyTorch: Excellent performance for dynamic neural networks and rapid prototyping
Computational Graphs
One of the fundamental differences between TensorFlow and PyTorch is how they handle computational graphs:
- TensorFlow: Originally used static computational graphs (define-and-run), though TensorFlow 2.0 now defaults to eager execution
- PyTorch: Uses dynamic computational graphs (define-by-run), allowing for more flexibility during development
In TensorFlow's original approach, the computational graph is generated statically before the code is run. This allows for parallelism and optimization but makes debugging more challenging. PyTorch's dynamic approach creates and executes graph nodes as you go, making it more intuitive and easier to debug.
Static graphs in TensorFlow are optimized for performance but can be less intuitive to debug. PyTorch's dynamic graphs make debugging easier as you can use standard Python debugging tools and see results immediately.
Debugging and Development
PyTorch has a significant advantage when it comes to debugging and development workflow. Since PyTorch operates eagerly and integrates seamlessly with Python, you can use standard Python debugging tools like pdb.
TensorFlow 2.0 has improved with eager execution, but its debugging experience still isn't as smooth as PyTorch's. However, TensorFlow offers excellent visualization tools like TensorBoard, which provides comprehensive insights into model training and performance.
Visualization Capabilities
When it comes to visualizing the training process, TensorFlow takes the lead with TensorBoard, which offers robust visualization capabilities:
- Tracking and visualizing metrics such as loss and accuracy
- Visualizing the computational graph (ops and layers)
- Viewing histograms of weights, biases, or other tensors as they change over time
- Displaying images, text, and audio data
- Profiling TensorFlow programs
PyTorch developers typically use Visdom, which offers more limited features like handling callbacks, plotting graphs, and managing environments. While functional, it doesn't match the comprehensive visualization capabilities of TensorBoard.
Model Deployment
TensorFlow offers more deployment options, including TensorFlow Serving, TensorFlow Lite, and TensorFlow.js, making it suitable for various platforms and devices. PyTorch, while more limited in deployment options, is catching up with tools like TorchServe.
When it comes to deploying trained models to production, TensorFlow has traditionally been the clear winner. TensorFlow Serving provides a framework that uses REST Client API for production deployment. PyTorch has improved its deployment capabilities in recent versions but still requires additional frameworks like Flask or Django for web deployment.
Deployment capabilities:
- TensorFlow:
- TensorFlow Serving for high-performance serving
- TensorFlow Lite for mobile and edge devices
- TensorFlow.js for browser-based ML
- TensorFlow Extended (TFX) for production ML pipelines
- PyTorch:
- TorchServe for model serving
- PyTorch Mobile for mobile deployment
- ONNX integration for cross-framework compatibility
Distributed Training
Both frameworks support distributed training, but with different approaches:
PyTorch optimizes performance by taking advantage of native support for asynchronous execution from Python. Its data parallelism feature makes it relatively simple to implement distributed training.
TensorFlow requires more manual configuration to run operations on specific devices for distributed training. While it's possible to replicate PyTorch's functionality in TensorFlow, it typically requires more effort and code.
Community and Ecosystem
Both TensorFlow and PyTorch have strong community support and a rich ecosystem of tools and libraries. TensorFlow's ecosystem is more extensive, with a wide range of pre-trained models and deployment options.
TensorFlow has a larger user base and more extensive documentation, with strong support from Google. PyTorch has gained significant traction in the research community, with many recent papers implementing their models in PyTorch first.
Key ecosystem components:
- TensorFlow: TensorFlow Hub, TensorFlow Datasets, TF-Agents for reinforcement learning
- PyTorch: torchvision, torchaudio, torchtext, PyTorch Lightning for simplified training
Industry Adoption
TensorFlow has historically had stronger industry adoption, particularly in production environments. Companies like Google, Airbnb, and Twitter use TensorFlow extensively in their production systems.
PyTorch has been gaining ground, especially in research-focused organizations and AI labs. Facebook, Tesla, and OpenAI are notable users of PyTorch. In fact, OpenAI standardized on PyTorch for its deep learning framework as of 2020, and it's likely that systems like ChatGPT use PyTorch as their primary machine learning framework.
Notable projects built with these frameworks include:
- PyTorch Projects: CheXNet (radiologist-level pneumonia detection), PYRO (probabilistic programming language)
- TensorFlow Projects: Magenta (creative machine learning), Sonnet (neural network building), Ludwig (code-free deep learning)
Code Examples
Here's a simple neural network implementation in both frameworks to illustrate their syntax differences:
PyTorch Example:
import torch
import torch.nn as nn
import torch.optim as optim
# Define a simple neural network
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(784, 128)
self.relu = nn.ReLU()
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
return x
# Create model, loss function, and optimizer
model = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01)
# Training loop (simplified)
def train(model, x_data, y_data):
model.train()
optimizer.zero_grad()
outputs = model(x_data)
loss = criterion(outputs, y_data)
loss.backward()
optimizer.step()
return loss.item()
TensorFlow/Keras Example:
import tensorflow as tf
from tensorflow.keras import layers, models
# Define a simple neural network
model = models.Sequential([
layers.Dense(128, activation='relu', input_shape=(784,)),
layers.Dense(10)
])
# Compile the model
model.compile(
optimizer=tf.keras.optimizers.SGD(0.01),
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy']
)
# Training (simplified)
def train(model, x_data, y_data):
history = model.fit(
x_data, y_data,
epochs=1,
batch_size=32,
verbose=0
)
return history.history['loss'][0]
As you can see, PyTorch follows an object-oriented approach more similar to traditional Python programming, while TensorFlow with Keras offers a more abstracted, high-level API.
Installation Guide
Both frameworks are continuously updated with new features to make training more efficient and powerful. Here's how to install the latest versions:
PyTorch Installation:
# Linux
pip3 install torch torchvision torchaudio --index-url https://download.pytorch.org/whl/cpu
# macOS
pip3 install torch torchvision torchaudio
# Windows
pip3 install torch torchvision torchaudio
TensorFlow Installation:
# Linux
python3 -m pip install tensorflow[and-cuda]
# macOS
python3 -m pip install tensorflow
# Windows Native (for versions < 2.11 with GPU support)
conda install -c conda-forge cudatoolkit=11.2 cudnn=8.1.0
python -m pip install "tensorflow<2.11"
# Windows WSL 2
python3 -m pip install tensorflow[and-cuda]
Conclusion
The choice between TensorFlow and PyTorch depends on your specific project requirements and preferences. Both frameworks are powerful and capable, and understanding their differences can help you make an informed decision.
Consider TensorFlow if:
- You need robust production deployment options
- You're working on a project that requires mobile or web deployment
- You prefer a higher-level API with less boilerplate code
- You need strong visualization tools like TensorBoard
- You're building large-scale, production-grade machine learning systems
Consider PyTorch if:
- You're working on research or prototyping
- You prefer a more Pythonic, intuitive interface
- You need dynamic computational graphs for your models
- You value easier debugging and a more straightforward development workflow
- You're already familiar with Python and want a framework that feels natural
Many data scientists and ML engineers learn both frameworks to leverage their respective strengths for different projects. As the field evolves, both frameworks continue to improve and adopt features from each other, making the choice less critical than it once was.
Need Expert Help With Your Project?
Our team of specialists is ready to help you implement the strategies discussed in this article and address your specific business challenges.